Computer Programs – coding:
The process of translating the algorithm into syntax of a given language is known as “Coding”. Since algorithm cannot be executed directly by the computer, it has to betranslated into a programming language.
Computer programs, known as software, are instructions that tell a computer what to do.A computer program is composed of data and instructions.The computer stores the data temporarily memory. The instructions in a computer program are like the steps in analgorithm. In a programming language like JavaScript, theinstructions are called statements.
Let us take a problem, collect its requirement, design the solution, implement it in Javascript and then test our program.
Problem Statement: To develop an automatic system that accepts marks of a student 4 subjects and generates his/her grade based on average marks.
Requirements Analysis Ask the users to enlist the rules for assigning grades. These rules are:
Average Marks | Grade |
>75 | Distinction |
60-75 | First Class |
50-60 | Second Class |
40-50 | Third Class |
<40 | No Class Alloted |
Design In this phase, write an algorithm that gives a solution to the problem.
Step 1: Get the 4 subjects marks
Step 2: Calculate total marks of 4 subjects.
Step 3: Calculate average amarks (ave) = total marks /4
Step 4: If ave >75 then print "Distinction"
Step 5: Else If ave >= 60 and ave <75 then print "First Class"
Step 6: Else If ave >= 50 and ave <60 then print "Second Class"
Step 7: Else If ave >= 40 and ave <50 then print "Third Class" else print "No class Alooted"
Step 6: End
Flow chart:
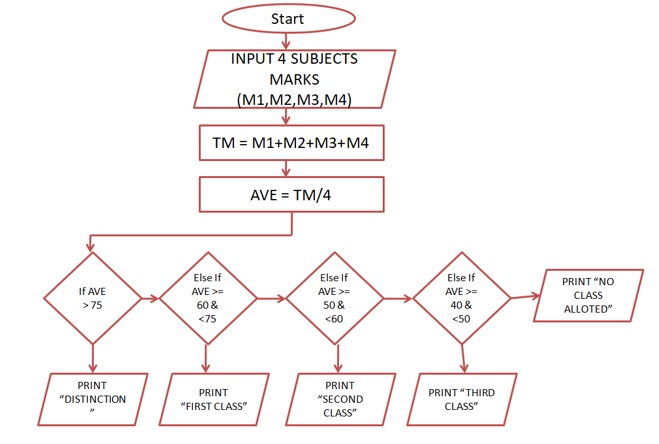
Implementation Write the Javascript program to implement the proposed algorithm.
let maths = parseFloat(prompt("Enter maths subject marks"));
let science = parseFloat(prompt("Enter science subject marks"));
let computer = parseFloat(prompt("Enter computer subject marks"));
let english = parseFloat(prompt("Enter english subject marks"));
let totalMarks = maths + science + computer + english;
let averageMarks = (totalMarks)/4;
if(averageMarks >= 75){
alert("Distinction");
}else if((averageMarks >= 60) && (averageMarks <75)){
alert("First Class");
}else if((averageMarks >= 50) && (averageMarks <60)){
alert("Second Class");
}else if((averageMarks >= 40) && (averageMarks <50)){
alert("Third Class");
}else{
alert("No class Alloted");
}
Test The above program is then tested with different test data to ensure that the program gives correct output for all relevant and possible inputs. The test cases are shown in the table given below.
Sl.No | Subjects marks | Average Marks | Expected Output | Actual Output |
1 | 75,76,75,76 | 77.5 | Distinction | Distinction |
2 | 55,65,68,75 | 65.75 | First Class | First Class |
3 | 58,55,48,65 | 56.5 | Second Class | Second Class |