Introduction
p5.js is a JavaScript library that simplifies the process of coding for the web, enabling users to create interactive graphics, animations, and complex visual compositions with ease.
One of the primary goals of p5js is to make it as easy as possible for beginners to learn how to program interactive, graphical applications.
Environment
First, you’ll need to get a code editor. A code editor is similar to a text editor (like Notepad or Notepad++), except it has special functionality for editing code instead of plain text. You can use any code editor you like.
There is also an official p5.js editor in development. If you would like to use it, you can download it by visiting https://editor.p5js.org/
Download and File Setup
Start by visiting https://p5js.org/download/ and selecting "p5.js complete." Double-click the .zip file that downloads, and drag the folder inside to a location on your hard disk. It could be Program Files or Documents or simply the desktop, but the important thing is for the p5 folder to be pulled out of that .zip file.
The p5 folder contains an example project that you can begin working from. Open your code editor. Next, you’ll want to open the folder named empty-example in your code editor. In most code editors, you can do this by going to the File menu in your editor and choosing Open..., then selecting the folder empty-example. You’re now all set up and ready to begin your first program!
First Program
When you open the empty-example folder, you will likely see a sidebar with the folder name at the top, and a list of the files contained in the folder directly below. If you click each of these files, you will see the contents of the file appear in the main area.
A p5.js sketch is made from a few different languages used together. HTML (HyperText Markup Language) provides the backbone, linking all the other elements together in a page. JavaScript (and the p5.js library) enable you to create interactive graphics that display on your HTML page. Sometimes CSS (Cascading Style Sheets) are used to further style elements on the HTML page, but we won’t cover that in this book.
If you look at the index.html file, you’ll notice that there is some HTML code there. This file provides the structure for your project, linking together the p5.js library, and another file called sketch.js, which is where you will write your own program.
The code that creates these links look like this:
<script language="javascript" type="text/javascript" src="../p5.js"></script>
<script language="javascript" type="text/javascript" src="sketch.js"></script>
You don’t need to do anything with the HTML file at this point—it’s all set up for you. Next, click sketch.js and take a look at the code:
function setup() { // put setup code here } function draw() { // put drawing code here }
The template code contains two blocks, or functions, setup() and draw().
setup() function is called automatically one time to configure the drawing canvas
draw() function is called automatically over and over again to update the canvas area (~60 times per second). This canvas "refresh" speed is called a frame rate, measured in frames per second (fps).
setup() contains instructions for setting up the environment, like canvas size, background color.
draw() contains instructions for drawing of lines, shapes, dots etc.
Our First Shape - A Circle: Try It
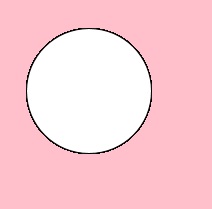
function setup() { createCanvas (300, 300); background('pink'); } function draw() { ellipse(100,100,125); }