Color
Color is defined as a single grayscale value, an RGB-value or an RGBA-value. Where each element has a range from 0 to 255.
The difference between the aforementioned values is as follows:
● A single grayscale value basically stands for a shade on a gradient from black to white. For greyscale, we have simply been providing one integer value (0 to 255) in our code.
● RGB contains three grayscale values which stand form Red, Green and Blue respectively: an RGB value with elements (255, 0, 0) would result in a bright red color, a value with elements (0, 255, 0) would result in a bright green color, and a value with elements (0, 0, 255) would result in a bright red color. Just like in Processing.
● RGBA adds an Alpha value to the RGB spectrum. This value stands for the opacity of the color. The alpha value you specify must also be in the range 0 (fully transparent) to 255 (fully opaque).
Gray Scale:
fill(25);
Program:Try It
function setup() { createCanvas(400, 400); } function draw() { // white background background(255,255,255); // Do not show outline noStroke() // Light grey fill(192); ellipse(100, 100, 100,100); // Medium Grey fill(128); ellipse(200, 200, 100, 100); //Dark Gray fill(64) ellipse(300, 100, 100, 100) // Black fill(0) ellipse(200, 300, 100, 100) }
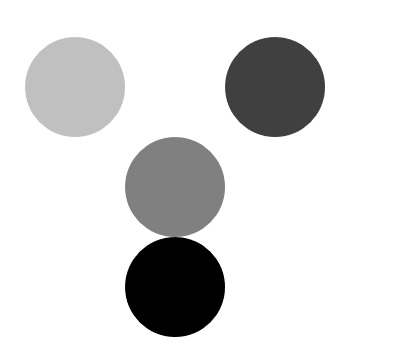
RGB Color:
fill(0,0,255);
Program:Try It
function setup() { createCanvas(400, 400); } function draw() { // white background background(255,255,255); // Do not show outline noStroke() // Light grey // Red fill(255, 0, 0) ellipse(100, 100, 100,100) // Green fill(0, 255, 0) ellipse(200, 200, 100, 100) // Blue fill(0, 0, 255) ellipse(300, 100, 100, 100) // "Dark Sea Green" fill(143, 188, 143) ellipse(200, 300, 100, 100) }
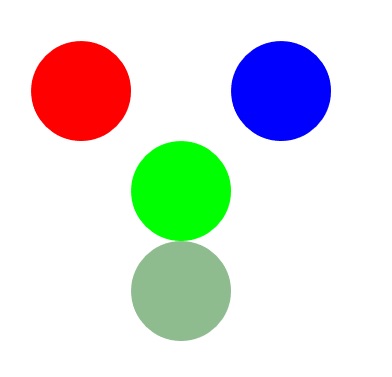
Colour Transparency:
fill(0,0,255,100);
Program:Try It
function setup() { createCanvas(400, 400); } function draw() { // white background background(255,255,255); // Do not show outline noStroke() // Light grey // Red fill(255, 0, 0, 100) ellipse(100, 100, 100,100) // Green fill(0, 255, 0, 100) ellipse(200, 200, 100, 100) // Blue fill(0, 0, 255, 100) ellipse(300, 100, 100, 100) // "Dark Sea Green" fill(143, 188, 143, 100) ellipse(200, 300, 100, 100) }
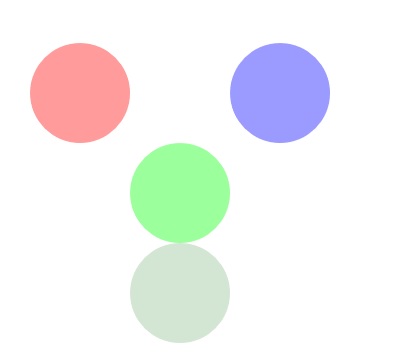
Changing background Color:
fill("pink");
Program:Try It
function setup() { createCanvas (400, 400); } function draw () { background(150, 150, 220); fill(200, 30, 255); ellipse(100, 100, 50, 50); }
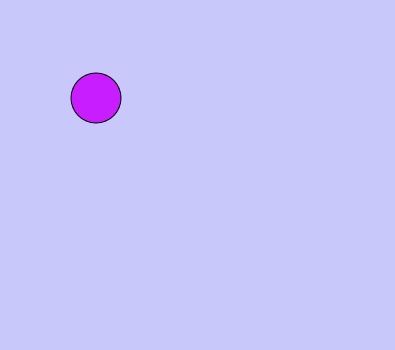
Changing Outline Stroke:
stroke('red');
Program:Try It
function setup() { createCanvas (400, 400); background('pink'); } function draw () { stroke('red'); fill('lightgreen'); ellipse(200, 200, 150); }
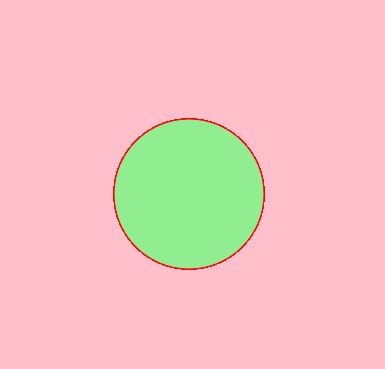
Setting stroke width:
strokeWeight(10);
Program:Try It
function setup() { createCanvas (400, 400); background('white'); } function draw () { stroke('red'); fill('lightgreen'); strokeWeight(5); ellipse(200, 200, 150); }
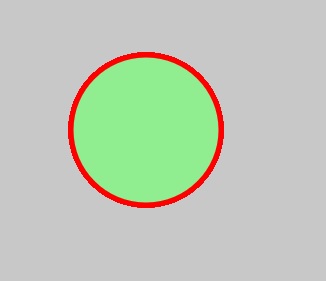