System Variables and RandomNumbers
System Variables
System variables: are special variables that are created and updated automaticallyby p5.js for you, so you do not need to declare them using the let keyword, or assign values to them using the assignment ( = ) operator.
System variables related to mousecoordinates:
mouseX – Current horizontal location of the mouse
mouseY – Current vertical location of the mouse
pmouseX – Previous horizontal location of the mouse
pmouseY – Previous vertical location of the mouse
Example Programs
Track the Mouse
function setup() { createCanvas(400, 400); fill(0, 102, 200); noStroke(); } function draw() { ellipse(mouseX, mouseY, 9, 9); }
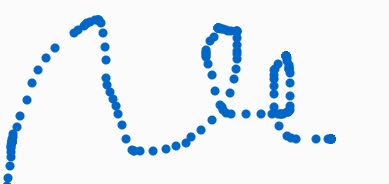
The Dot Follows You
function setup() { createCanvas(400, 400); fill(0, 102, 200); noStroke(); } function draw() { background(204); ellipse(mouseX, mouseY, 9, 9); }
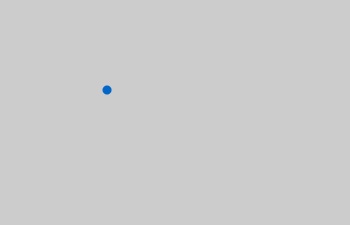
Draw Continuously
function setup() { createCanvas(400, 400); strokeWeight(4); stroke(0, 100,200); } function draw() { line(mouseX, mouseY, pmouseX, pmouseY); }
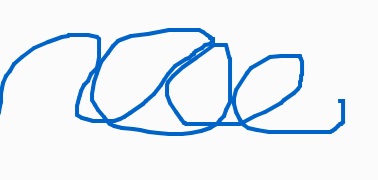
Set Thickness on the Fly
function setup() { createCanvas(600, 600); stroke(200, 200, 2); } function draw() { var weight = dist(mouseX, mouseY, pmouseX, pmouseY); strokeWeight(weight); line(mouseX, mouseY, pmouseX, pmouseY); }
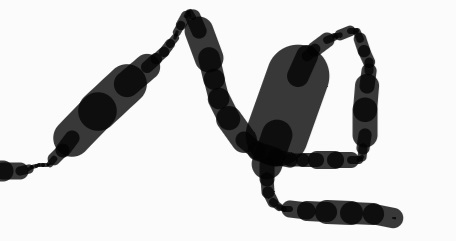
Other system variables
width – Width (in pixels) of canvas
height – Height (in pixels) of canvas
frameCount – Number of frames processed
frameRate () – When called with no parameters returns the current fps
mouseIsPressed – true or false: Is a mouse button pressed?
mouseButton – Which button is pressed? LEFT, RIGHT, or CENTER
key – Most recent key pressed (character) on the keyboard
keyCode – Numeric code (ASCII value) for the key pressed on the keyboard
keyIsPressed – true or false: Is a key pressed?
Example Program
Change the color by frame count
function setup() { createCanvas(400, 400); alert('Canvas is '+width+' x '+height+' px'); } function draw() { background(220); console.log(frameCount); fill(frameCount%256); rectMode(CENTER); rect(width/2,height/2,mouseX,mouseY); }
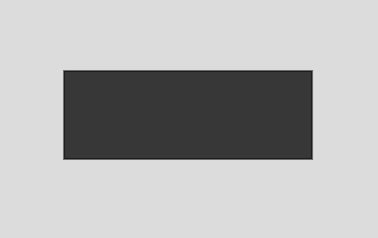
Random Numbers
P5.js random() function returns a randomly chosen value between 0 up to 1, excluding 1. You can also tell the function on what range to choose a number from or you can give it an set of numbers (an array) and it will choose one of the given values.
random(); // returns a value from 0 up to 1
random(max); // returns a value from 0 up to max
random(min , max); // returns a value from min up to max
random(choices); // returns one of the choices that are given in an array
Example Programs
Fill the Canvas with circles
function setup () { createCanvas ( 400 , 400 ); background (220); } function draw () { x = random ( 0 , width ); y = random ( 0 , height ); fill ( 10 , 30 , 175 ); ellipse ( x , y , 50 , 50 ); }
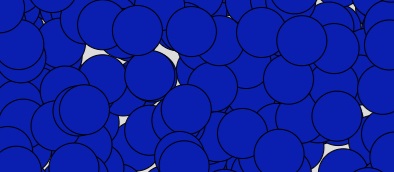
Fill the Canvas with color circles
function setup() { createCanvas(400,400); } function draw() { fill(random(350),random(350), random(150)); circle(random(350),random(350), random(150)); }
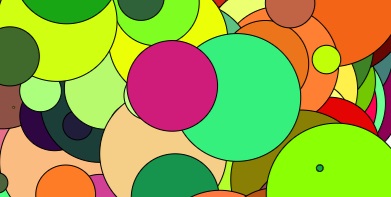
Try Your self
1. Diameter of the circle grows and shrinks depending on the distance of the mouse pointer to the center of the circle. Hints: You’ll need the dist() function.
dist() function is used to calculate the distance between the two points. syntex: dist(x1, y1, x2, y2);
var dia = 1; function setup() { createCanvas(400,400); } function draw() { circle(width/2, height/2, dia); dia = dist(0,0,mouseX,mouseY); }
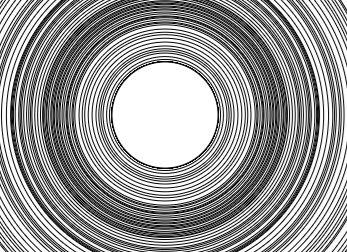
2. Move the following circles to the center according to the mouse pointer.
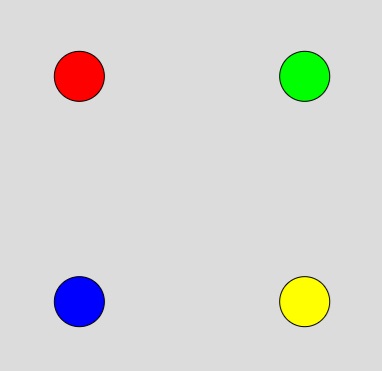
var speed = 1; var change = 1; function setup() { createCanvas(400,400); } function draw() { background(220); fill(255,0,0); ellipse(50+change, 50+change, 50, 50); fill(0,255,0); ellipse(350-change, 50+change, 50, 50); fill(0,0,255); ellipse(50+change, 350-change, 50, 50); fill(255,255,0); ellipse(350-change, 350-change, 50, 50); change = dist(0,0,mouseX/2,mouseY/2); }
3. Write a program that draws a single line(). One end of the line should always start at the bottom-left of the canvas (0,400) and the other should follow the mouse pointer.
function setup() { createCanvas(400,400); } function draw() { background(220); line(0,400, mouseX,mouseY); }
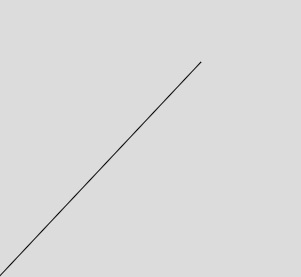
4. Modify the program above, such that you draw 4 lines. Each line should have an end attached to one of the four corners of the canvas and the other end following the mouse. Try hiding the mouse pointer this time using noCursor() function.
function setup() { createCanvas(400,400); } function draw() { noCursor(); background(220); line(0,0, mouseX,mouseY); line(400,0, mouseX,mouseY); line(0,400, mouseX,mouseY); line(400,400, mouseX,mouseY); }
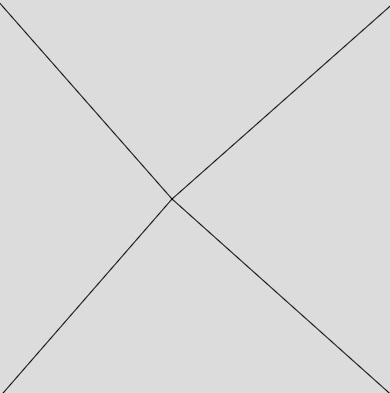
5. Write a program that draws four differently colored triangles on the screen. The base of each triangle should be one of the four edges of the screen, and one point of each triangle should follow the mouse pointer. Their bases always stay attached to the screen edges.
Give each triangle a different fill colour. Can you do this by only drawing 3 triangles?
Try hand mouse pointer this time using cursor(HAND) function
(also: ARROW, HAND, MOVE, TEXT, or WAIT)
function setup() { createCanvas(400,400); } function draw() { cursor(HAND); background("green"); fill("yellow"); triangle(0,0,400,0,mouseX,mouseY); fill("red"); triangle(0,0,0,400,mouseX,mouseY); fill("blue"); triangle(0,400,400,400,mouseX,mouseY); }
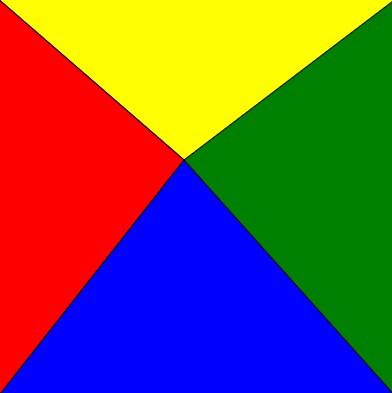