Selection or Control statements (Decision Making)
Selection statements, or control statements are used to allow a computer to make choices.A program can decide which statements to execute based on a condition.
One-Way Selection Statements (if statement)
The simplest form of selection is the if statement. This type of control statement is also called a one-way selection statement, because it consists of a condition and just a single sequence of statements. If the condition is True, the sequence of statements is run. Otherwise, control proceeds to the next statement following the entire selection statement.
Syntax for the if statement:
if condition: sequenceƒof statements
Simple if statements are often used to prevent an action from being performed if a condition is not right.
Example:
age = int(input("Enter your age ")) if age >= 18: print("Eligible to vote")
Flow chart of if statements:
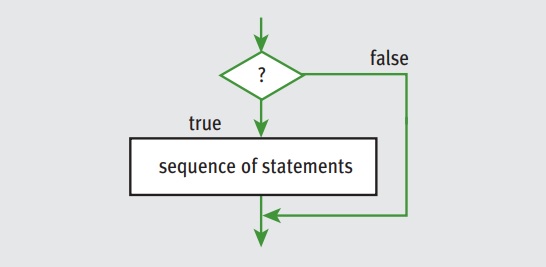
Two-way selection statement (if-else Statements)
The if-else statement is the most common type of selection statement. It is also called a two-way selection statement, because it directs the computer to make a choice between two alternative courses of action.
The if-else statement is often used to check inputs for errors and to respond with error messages if necessary. The alternative is to go ahead and perform the computation if the inputs are valid.
The flowchart of the if-else statement
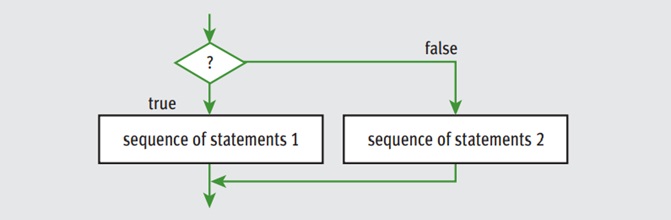
Syntax for if..else statement is as follows
if condition: statement(s) else: statement(s)
Example:
age = int(input("Enter your age: ")) if age >= 18: print("Eligible to vote") else: print("Not eligible to vote")
Example:
Import math area=float(input("Enter the area:")) if area > 0: radius = math.sqrt(area / math.pi) print("The radius is", radius) else: print("Error: the area must be a positive number")
Multi-Way if Statements (if..elif: statements):
Many a times there are situations that require multiple conditions to be checked and it may lead to many alternatives. In such cases we can chain the conditions using if..elif
The syntax for a selection structure using elif is as follows:
if condition: statement(s) elif condition: statement(s) elif condition: statement(s) else: statement(s)
Example:
number = int(input("Enter a number: ") if number > 0: print("Number is positive") elif number < 0: print("Number is negative") else: print("Number is zero")
Example Programs
1.Write a Python program to get the three test scores, calculate their average test score and congratulate if the average is greater than 95.
# define HIGH_SCORE constant HIGH_SCORE = 95 # Get the three test scores test1 = float(input('Enter the score for test 1: ')) test2 = float(input('Enter the score for test 2: ')) test3 = float(input('Enter the score for test 3: ')) # Calculate the average test score. average = (test1 + test2 + test3) / 3 # Print the average. print('The average score is', round(average,2)) # If the average is a high score, # congratulate the user. if average >= HIGH_SCORE: print('Congratulations!') print('That is a great average!') OUTPUT: Enter the score for test 1: 96.5 Enter the score for test 2: 97.0 Enter the score for test 3: 98.0 The average score is 97.17 Congratulations! That is a great average!
2.Write a Python program to find the larger of two numbers input by the user.
# Obtain the two numbers from the user. num1 = eval(input("Enter the first number: ")) num2 = eval(input("Enter the second number: ")) # Determine and display the larger value. if num1 > num2: largerValue = num1 else: largerValue = num2 print("The larger value is", largerValue, ".") #print("The larger value is", str(largerValue) + ".") OUTPUT: Enter the first number: 65 Enter the second number: 85 The larger value is 85.
3.Write a Python program to Find the largest of three numbers.
# Input the three numbers. firstNumber = eval(input("Enter first number: ")) secondNumber = eval(input("Enter second number: ")) thirdNumber = eval(input("Enter third number: ")) # Determine and display the largest value. max = firstNumber if secondNumber > max: max = secondNumber if thirdNumber > max: max = thirdNumber print("The largest number is", str(max) + ".") OUTPUT: Enter first number: 52 Enter second number: 85 Enter third number: 95 The largest number is 95.
4.Write a program to find the grade of a student when grades are allocated as A - Above 90%, B - 80% to 90%, C - 70% to 80%, D - 60% to 70% and E -Below 60%. Percentage of the marks obtained by the student is input to the program.
#Program to print the grade of the student n = float(input('Enter the percentage of the student: ')) if(n > 90): print("Grade A") elif(n > 80): print("Grade B") elif(n > 70): print("Grade C") elif(n >= 60): print("Grade D") else: print("Grade E") OUTPUT: Enter the percentage of the student: 82 Grade B
5.Write a program that interprets the Body Mass Index (BMI) based on a user's weight and height.
It should tell them the interpretation of their BMI based on the BMI value.
• Under 18.5 they are underweight
• Over 18.5 but below 25 they have a normal weight
• Over 25 but below 30 they are slightly overweight
• Over 30 but below 35 they are obese
• Above 35 they are clinically obese.
# Input the height and weight. height = float(input("Enter your height in m: ")) weight = float(input("Enter your weight in kg: ")) # calculate bmi. bmi = round(weight / height ** 2) # Tell the interpretation if bmi < 18.5: print(f"Your BMI is {bmi}, you are underweight.") elif bmi < 25: print(f"Your BMI is {bmi}, you have a normal weight.") elif bmi < 30: print(f"Your BMI is {bmi}, you are slightly overweight.") elif bmi < 35: print(f"Your BMI is {bmi}, you are obese.") else: print(f"Your BMI is {bmi}, you are clinically obese.") OUTPUT: Enter your height in m: 1.72 Enter your weight in kg: 55 Your BMI is 19, you have a normal weight.
6.Write a program that works out whether if a given year is a leap year.
A year is a leap year if it is divisible by 4 but not by 100 or if it is divisible by 400.
year = eval(input("Enter a year: ")) if year%4 == 0: if year % 100 == 0: if year % 400 == 0: print(year, "is a leap year") else: print(year, "is not a leap year") else: print(year, "is a leap year") else: print(year, "is not a leap year") OUTPUT: Enter a year: 2002 2002 is not a leap year
year = eval(input("Enter a year: ")) # Check if the year is a leap year isLeapYear = ((year % 4 == 0 and year % 100 != 0 ) or (year % 4 == 0)) # Display the result print(year, "is a leap year?", isLeapYear) OUTPUT: Enter a year: 2002 2002 is a leap year? False
7.Write a program to Addition quiz using random numbers.
import random # Generate random numbers number1 = random.randint(0, 9) number2 = random.randint(0, 9) # Prompt the user to enter an answer answer = eval(input("What is " + str(number1) + " + " + str(number2) + " ? ")) if answer == number1 + number2: print("Correct! " + str(number1) + " + " + str(number2) + " = ", number1 + number2 ) else: print("Wrong! " + str(number1) + " + " + str(number2) + " = ", number1 + number2) OUTPUT: What is 3 + 4 ? 7 Correct! 3 + 4 = 7