Working with Python
To write and run (execute) a Python program, we need to have a Python interpreter installed on our computer or we can use any online Python interpreter. The interpreter is also called Python shell.
Installing Python:
Go to www.python.org/downloads/ and download the latest version of Python.
The Python Interpreter:
The Python interpreter is a program that can read Python programming statements and execute them.
Interpreter Execution Modes
There are two ways to use the Python interpreter:
a) Interactive mode
b) Script mode
In interactive mode, the interpreter waits for you to type Python statements on the keyboard. Once you type a statement, the interpreter executes it and then waits for you to type another statement.
In script mode, the interpreter reads the contents of a file that contains Python statements. Such a file is known as a Python program or a Python script. The interpreter executes each statement in the Python program as it reads it.
Interactive Mode:
Once Python has been installed and set up on your system, you start the interpreter in interactive mode by going to the operating system’s command line and typing the following command:
python
If you are using Windows, you can alternatively type python in the Windows search box. In the search results, you will see a program named something like Python 3.5. (The “ 3.5” is the version of Python that is installed. At the time this is being written, Python 3.5 is the latest version.) Clicking this item will start the Python interpreter in interactive mode.
NOTE: When the Python interpreter is running in interactive mode, it is commonly called the Python shell.
When the Python interpreter starts in interactive mode, you will see something like the following displayed in a console window:
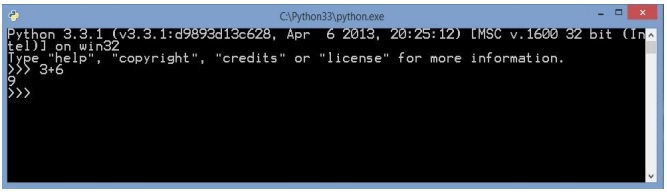
The >>> that you see is a prompt that indicates the interpreter is waiting for you to type a Python statement.
Let’s try it out. One of the simplest things that you can do in Python is print a message on the screen. For example, the following statement prints the message Python programming is fun! on the screen:
print('Python programming is fun!')
To run a python file on command line:
exec(open("C:\Python33\python programs\program1.py").read( ))
Writing Python Programs and Running Them in Script Mode
Although interactive mode is useful for testing code, the statements that you enter in interactive mode are not saved as a program. They are simply executed and their results displayed on the screen. If you want to save a set of Python statements as a program, you save those statements in a file. Then, to execute the program, you use the Python interpreter in script mode.
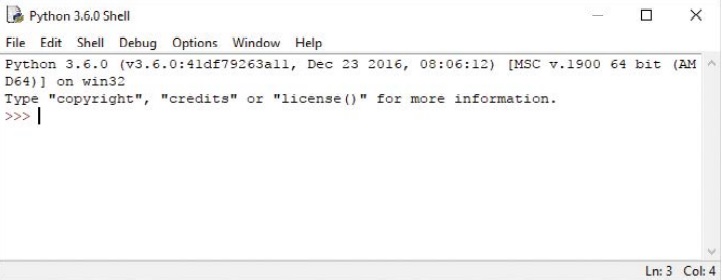
For example, suppose you want to write a Python program that displays the following two lines of text:
Welcome to python programming. Python programming is fun!
Write the following lines of code in a simple text editor like notepad and save with .py extention (test.py)
print(Welcome to python programming.) print(Python programming is fun!)
To run the program, you would go to the directory in which the file is saved and type the following command at the operating system command line:
python test.py
This starts the Python interpreter in script mode and causes it to execute the statements in the file test.py. When the program finishes executing, the Python interpreter exits.
The IDLE Programming Environment
An integrated development environment is a single program that gives you all of the tools you need to write, execute, and test a program.
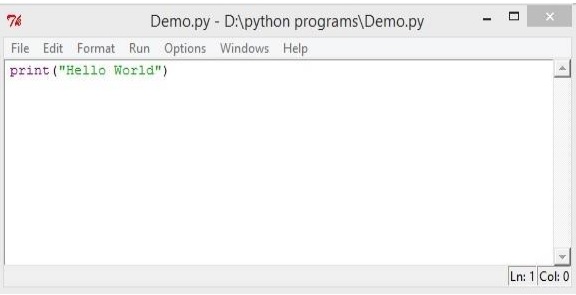
Recent versions of Python include a program named IDLE, which is automatically installed when the Python language is installed. (IDLE stands for Integrated DeveLopment Environment.) The >>> prompt appears in the IDLE window, indicating that the interpreter is running in interactive mode. You can type Python statements at this prompt and see them executed in the IDLE window.
IDLE also has a built-in text editor with features specifically designed to help you write Python programs.
A first program
Start IDLE and open up a new window (choose New Window under the File Menu). Type in the following program.
temp = eval(input('Enter a temperature in Celsius: ')) print('In Fahrenheit, that is', 9/5*temp+32)
Then, under the Run menu, choose Run Module (or press F5). IDLE will ask you to save the file, and you should do so. Be sure to append .py to the filename as IDLE will not automatically append it. This will tell IDLE to use colors to make your program easier to read.
Once you’ve saved the program, it will run in the shell window. The program will ask you for a temperature. Type in 20 and press enter. The program’s output looks something like this:
Enter a temperature in Celsius: 20 In Fahrenheit, that is 68.0