Turtle graphics
Turtle graphics is an interesting and easy way to learn basic programming concepts. The Python turtle graphics system simulates a "turtle" that obeys commands to draw simple graphics.
In the late 1960s, MIT professor Seymour Papert used a robotic "turtle" to teach programming. The turtle was tethered to a computer where a student could enter commands, causing the turtle to move. The turtle also had a pen that could be raised and lowered, so it could be placed on a sheet of paper and programmed to draw images.
Python has a turtle graphics system that simulates a robotic turtle. The system displays a small cursor (the turtle) on the screen. You can use Python statements to move the turtle around the screen, drawing lines and shapes.
The first step in using Python’s turtle graphics system is to write the following statement:
import turtle
This statement is necessary because the turtle graphics system is not built into the Python interpreter. Instead, it is stored in a file known as the turtle module. The import turtle statement loads the turtle module into memory so the Python interpreter can use it.
Turtle Graphics window
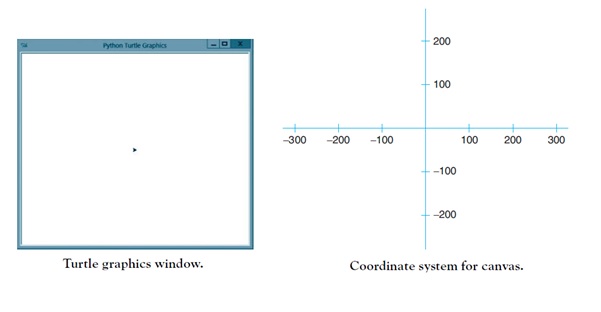
The white region inside the border is called the canvas and the small chevron in the center of the canvas is called a turtle. The canvas contains around 360,000 points called pixels that are identified by ordered pairs of numbers determined by the coordinate system in the following figure. The pixel in the center of the canvas has coordinates (0, 0).
Notice The turtle doesn’t look like a turtle at all. Instead, it looks like an arrowhead. This is important, because it points in the direction that the turtle is currently facing. If we instruct the turtle to move forward, it will move in the direction that the arrowhead is pointing.
Drawing Lines with the Turtle
When a turtle object is created, its position is set at (0, 0)—the center of the window—and its direction is set to go straight to the right. The turtle module uses a pen to draw shapes. By default, the pen is down (like the tip of an actual pen touching a sheet of paper). When you move the turtle, it draws a line from the current position to the new position if the pen is down.
Turning the Turtle
When the turtle first appears, its default heading is 0 degrees (East).
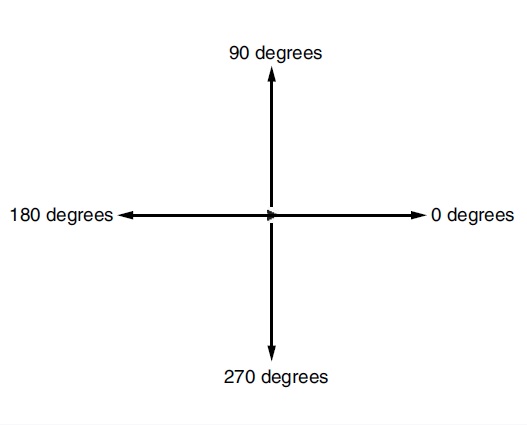
You can turn the turtle so it faces a different direction by using either the turtle. right(angle) command, or the turtle.left(angle) command. The turtle. right(angle) command turns the turtle right by angle degrees, and the turtle.left(angle) command turns the turtle left by angle degrees. Here is an example session that uses the turtle.right(angle) command:
Turning the Turtle right:
Example:
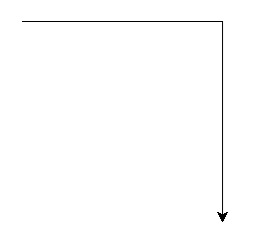
import turtle turtle.forward(200) turtle.right(90) turtle.forward(200)
Setting the Turtle’s Heading to a Specific Angle
You can use the turtle.setheading(angle) command to set the turtle’s heading to a specific angle. Simply specify the desired angle as the angle argument.
Setting the Turtle’s Heading to 45 deg.
Example:
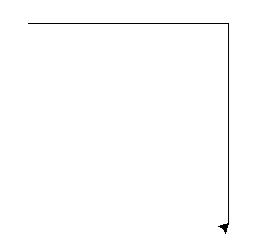
import turtle turtle.forward(200) turtle.right(90) turtle.forward(200) urtle.setheading(45)
Turtle Pen Drawing State Methods
turtle.pendown() Pulls the pen down—drawing when moving.
turtle.penup() Pulls the pen up—no drawing when moving.
turtle.pensize(width) Sets the line thickness to the specified width.
Turtle Motion Methods
turtle.forward(d) Moves the turtle forward by the specified distance in the direction the turtle is headed.
turtle.backward(d) Moves the turtle backward by the specified distance in the opposite direction the turtle is headed. The turtle’s direction is not changed.
turtle.right(angle) Turns the turtle right by the specified angle.
turtle.left(angle) Turns the turtle left by the specified angle.
turtle.goto(x, y) Moves the turtle to an absolute position.
turtle.setx(x) Moves the turtle’s x-coordinate to the specified position.
turtle.sety(y) Moves the turtle’s y-coordinate to the specified position.
turtle.setheading(angle) Sets the orientation of the turtle to a specified angle. 0-East, 90-North, 180-West, 270-South.
turtle.home() Moves the turtle to the origin (0, 0) and east direction.
turtle.circle(r, ext, step) Draws a circle with the specified radius, extent, and step.
turtle.dot(diameter, color) Draws a circle with the specified diameter and color.
turtle.undo() Undo (repeatedly) the last turtle action(s).
turtle.speed(s) Sets the turtle’s speed to an integer between 1 and 10, with 10 being the fastest.
Drawing Simple Shapes
Example:
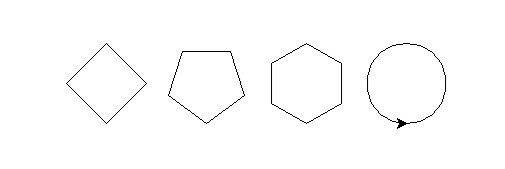
import turtle turtle.penup() turtle.goto(-100, -50) turtle.pendown() turtle.circle(40, steps = 4) # Draw a square turtle.penup() turtle.goto(0, -50) turtle.pendown() turtle.circle(40, steps = 5) # Draw a pentagon turtle.penup() turtle.goto(100, -50) turtle.pendown() turtle.circle(40, steps = 6) # Draw a hexagon turtle.penup() turtle.goto(200, -50) turtle.pendown() turtle.circle(40) # Draw a circle turtle.done()
Turtle Pen Color, Filling, and Drawing Methods
turtle.color(c) Sets the pen color.
turtle.fillcolor(c) Sets the pen fill color.
turtle.begin_fill() Calls this method before filling a shape.
turtle.end_fill() Fills the shapes drawn before the last call to begin_fill.
turtle.filling() Returns the fill state: True if filling, False if not filling.
turtle.clear() Clears the window. The state and the position of the turtle are not affected.
turtle.reset() Clears the window and reset the state and position to the original default value.
turtle.screensize(w, h) Sets the width and height of the canvas.
turtle.hideturtle() Makes the turtle invisible.
turtle.showturtle() Makes the turtle visible.
turtle.isvisible() Returns True if the turtle is visible.
turtle.write(s, font=("Arial",8, "normal")) Writes the string s on the turtle position. Font is a triple consisting of fontname, fontsize, and fonttype.
Filling Shapes and setting colors:
Example:
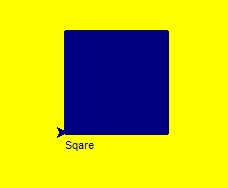
import turtle turtle.setup(640, 480) turtle.bgcolor(1.0,1.0,0) turtle.showturtle() turtle.penup() turtle.pensize(5) turtle.speed(10) turtle.color(0.0,0.0,0.5) turtle.goto(-60, 60) turtle.write("Sqare") turtle.goto(-60, 80) turtle.pendown() turtle.begin_fill() turtle.forward(100) turtle.left(90) turtle.forward(100) turtle.left(90) turtle.forward(100) turtle.left(90) turtle.forward(100) turtle.left(90) turtle.end_fill() turtle.done()
Drwaing a star:
Example:
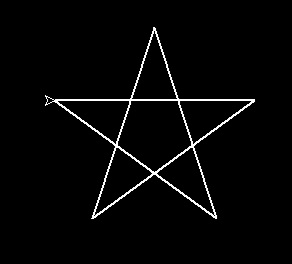
import turtle turtle.setup(640, 480) turtle.bgcolor("black") turtle.pensize(2) turtle.pencolor("white") for i in range(5): turtle.forward(200) turtle.right(144) turtle.done()
Drwaing a star (while loop):
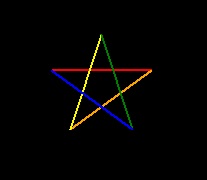
Example:
import turtle turtle.setup(640, 480) turtle.bgcolor("black") turtle.pensize(2) turtle.speed(10) colors = ["red", "orange", "yellow", "green", "blue", "purple"] count = 0 while count <= 4: turtle.pencolor(colors[count]) turtle.forward(100) turtle.right(144) count = count + 1 turtle.hideturtle() turtle.done()
Drwaing a star using a function:
Example:
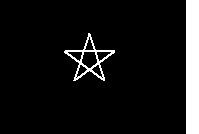
import turtle turtle.setup(640, 480) turtle.bgcolor("black") turtle.pensize(2) turtle.speed(5) def star(): for i in range(5): turtle.forward(50) turtle.right(144) turtle.color("white") star() turtle.hideturtle() turtle.done()
Drwaing a star at a random position:
Example:
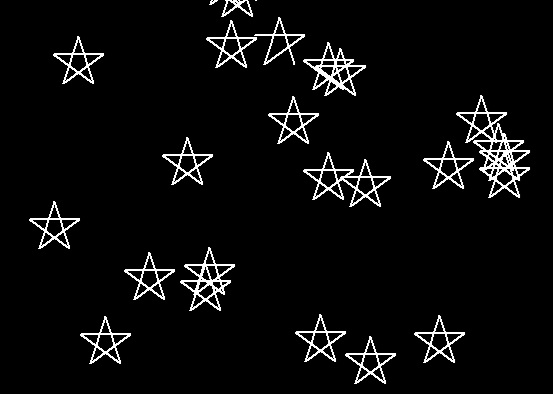
import turtle import random turtle.setup(640, 480) turtle.bgcolor("black") turtle.pensize(2) turtle.speed(10) turtle.penup() def star(): turtle.hideturtle() for i in range(5): turtle.forward(50) turtle.right(144) turtle.color("white") for i in range(100): x = random.randint(-250, 250) y = random.randint(-250, 250) turtle.goto(x,y) turtle.pendown() star() turtle.penup() turtle.hideturtle() turtle.done()
Clear screen after some count:
Example:
import turtle import random turtle.setup(640, 480) turtle.bgcolor("black") turtle.pensize(2) turtle.speed(10) turtle.penup() colors = ["red", "orange", "yellow", "green", "blue", "purple"] def star(): turtle.hideturtle() turtle.pencolor(colors[random.randint(0,5)]) for i in range(5): turtle.forward(50) turtle.right(144) turtle.color("white") i = 0 while i < 30: x = random.randint(-250, 250) y = random.randint(-250, 250) turtle.goto(x,y) turtle.pendown() star() turtle.penup() i = i +1 if i > 25: turtle.clear() i = 0 turtle.hideturtle() turtle.done()
Drawing basic shapes:
Example:
import turtle def triangle(length): for i in range(3): turtle.forward(length) turtle.right(120) def suare(length): for i in range(4): turtle.forward(length) turtle.right(90) def rect(w,h): for i in range(4): turtle.forward(w) turtle.right(90) turtle.forward(h) turtle.right(90) def star(w): for i in range(120): turtle.forward(w) turtle.right(176) turtle.fillcolor("red") turtle.color("red") #turtle.begin_fill() star(150) #turtle.end_fill() turtle.hideturtle() turtle.done()
Drwaing trigonometric functions:
Example:
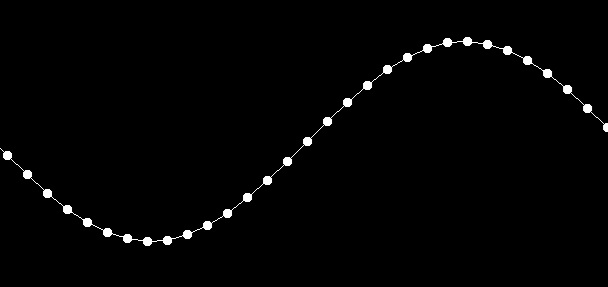
import turtle import math turtle.bgcolor("black") turtle.color("white") turtle.speed(10) turtle.penup() for x in range(-800,800,20): y = math.sin(x/100)*100 turtle.goto(x, y) turtle.pendown() turtle.dot(10) turtle.done()
Drwaing trigonometric functions:
Example:
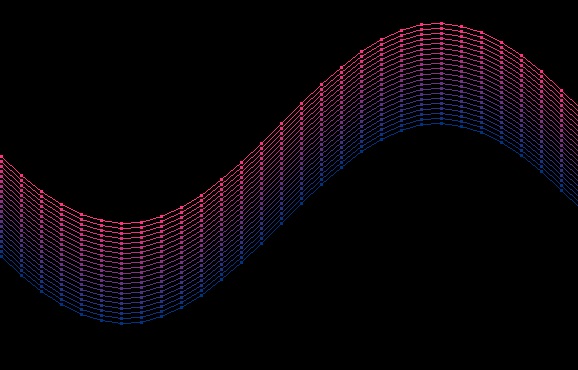
import turtle import math turtle.bgcolor("black") turtle.color("white") turtle.speed(10) turtle.penup() for step in range(0,500,5): for x in range(-500,500,20): y = math.sin(x/100)*100 turtle.goto(x, y+ step) turtle.color(step/100,0.2,0.5) turtle.pendown() turtle.dot(4) turtle.penup() turtle.done()
Turtle Grapics-1:
Example:
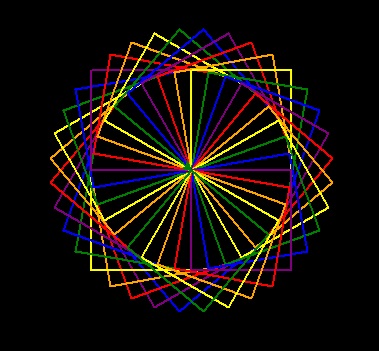
import turtle turtle.setup(640, 480) turtle.bgcolor("black") turtle.pensize(2) turtle.speed(10) turtle.penup() colors = ["red", "orange", "yellow", "green", "blue", "purple"] turtle.pendown() for i in range(0,40): turtle.color(colors[i%6]) turtle.right(10) for i in range(0,4): turtle.forward(100) turtle.right(90) turtle.done()
Turtle Grapics-2:
Example:
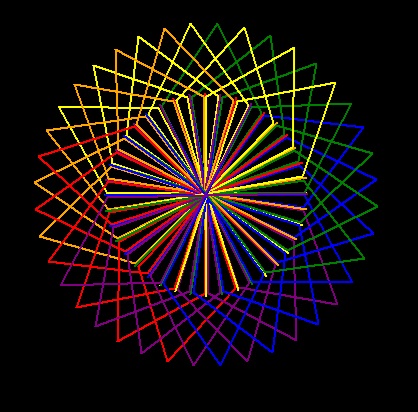
import turtle colors = ["red", "orange", "yellow", "green", "blue", "purple"] pen = turtle.Turtle() pen.speed(10) turtle.bgcolor("black") pen.pensize(2) for i in range(60): pen.color(colors[i % 6]) pen.forward(200) pen.right(61) pen.forward(100) pen.right(120) pen.forward(100) pen.right(61) pen.forward(200) pen.right(181) pen.hideturtle() turtle.done()
Turtle Grapics-3:
Example:
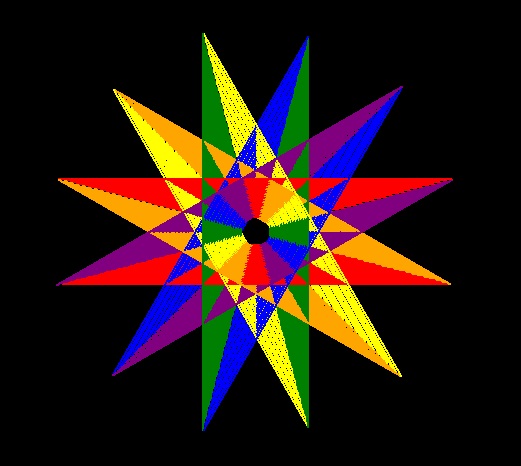
import turtle colors = ["red", "orange", "yellow", "green", "blue", "purple"] pen = turtle.Turtle() pen.speed(10) turtle.bgcolor("black") pen.pensize(2) initial_size = 100 for i in range(300): pen.color(colors[i % 6]) pen.forward(initial_size + i) pen.left(150) pen.hideturtle()