Variables and Strings
A collection of characters, such as the Hello, world! message, is called a String.
A variable is little more than a portion of memory that has been given a name.
When you define a variable, you must also tell the compiler what type of data is to be associated with that variable.
The data type of the variable is important because it determines how many bytes of memory are dedicated to that variable, and what kind of data can be stored in the variable.
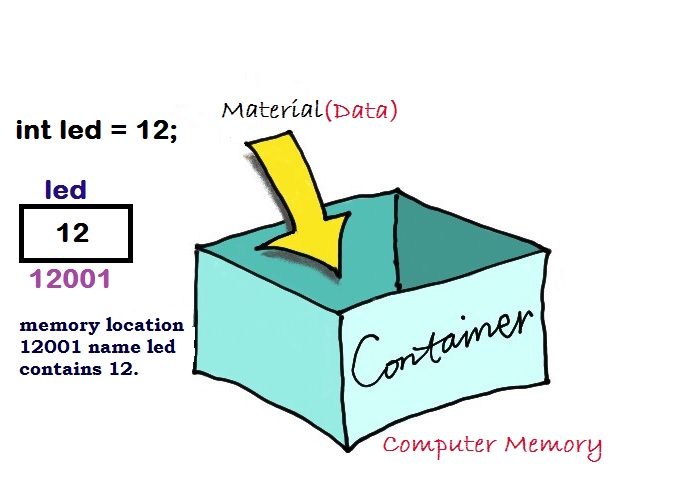
int ledPin = 13; // variable
void setup()
{
pinMode(ledPin, OUTPUT);
}
void loop()
{
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
Key Points:
Variables are used to represent values that may be changed in the program.
We can use a variable many times in a program.
Variables make it easier to store, read and change the data within the computer's memory by allowing you to associate easy-to-remember labels (increases program readability).
We can easily modify the variables. For example, if we want to change the value of variable ledPin from 13 to 8, we need to change the only at one point in the code (i.e. at pinMode declaration statement).
Keywords in C:
Each of the types shown in above (i.e., boolean, char, int, etc.) are keywords in C. A keyword is any word that has special meaning to the C compiler. Because keywords are reserved for the compiler’s use, you cannot use them for your own variable or function names. If you do, the compiler will flag it as an error. If the compiler didn’t flag such errors, then the compiler would be confused as to which use of the keywords to use in any given situation.
Variable Names in C:
If you can’t use keywords for variable or function names, then what can you use? There are three general rules for naming variables or functions in C.
Valid variable names may contain:
1. Characters a through z and A through Z
2. The underscore character (_)
3. Digit characters 0 through 9, provided they are not used as the first character in the
name.
Variable Declaration:
All variables have to be declared before they can be used. Declaring a variable
means defining its value type, as in int, float etc, setting a specified name, and
optionally assigning an initial value.
Example: int lightSignal;
Changing the value of a variable (Assignment):
The value of a variable can be changed using the assignment operator ( equal = sign).
But we need to declare a variable before assigning the value.
Example: ldrPin = A0;
lightSignal = analogRead(ldrPin);
Variable declaration and Initialisation in a single statement:
int lightSignal = analogRead(ldrPin);
Variable Scope: It means that in how many ways the variables can be declared. The variables can be declared in two ways in Arduino, which are listed below:
Local Variables: The local variables are declared within the function. The variables have scope only within the function. These variables can be used only by the statements that lie within that function.
Global Variables: The global variables can be accessed anywhere in the program. The global variable is declared outside the setup() and loop() function.
Arduino – Constants
Constants are pieces of information that do not change over time. Since they cannot be modified by the program, they provide stability and help to ensure that the code remains consistent. In Arduino programming, constants can be declared as integers, strings, or floating-point numbers (decimals). They must also have a type — such as const int or const float — assigned before they are used in Arduino functions or operations.
Constants are declared in a similar way but instead use the keyword “const” followed by its type, name, and value.
For example, to declare a constant named "myConstant" with value 6: const int myConstant = 6; This assigns the fixed value of 6 that cannot be changed during run-time.
Arduino – Data Types
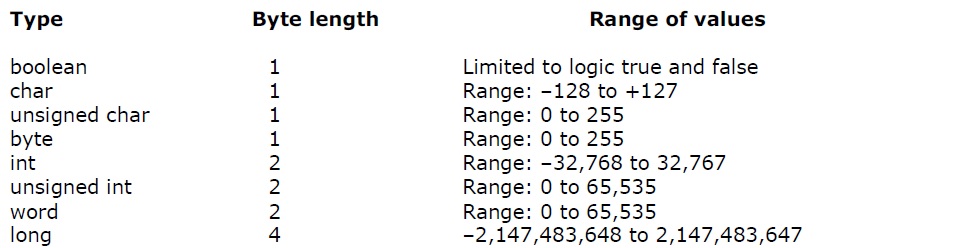
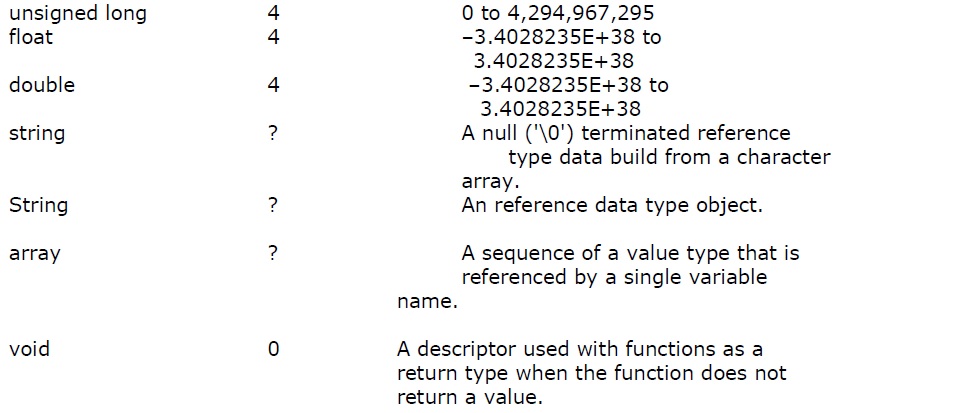
Learning Objectives:
1. Know that a variable is a name that can be assigned a value.
2. Be able to follow naming rules and conventions.
3. Know that before a variable can be used it must be declared.
4. Be able to declare variables.
5. Be able to declare variables and assign initial values as part of the declaration.
6. Know how to work with the String data type, including use of the concatenation operator +
Task List
Sl.no | Task and Reference |
---|---|
1 | Declare a String variable: String bird; |
2 | Add a String literal to the above variable: bird = "parrot"; |
3 | Add a String literal to the above variable: String message = bird + "can Fly"; |
4 | Declare two String variables: String str1, str2; |
5 | Assign values to the above String variables: str1 = "Hello"; |
6 | Concatenate str1 with a space and str2 to print hello world!: String str3 = str1 + " " + str2; |
Vocabulary
String A sequence of characters treated as one object. Example: “Hello, World!”.
variable A name given to a location in memory where a value can be stored. A variable is for a speciic type. The name must follow some naming rules. Putting a value into memory is referred to as assigning that value to the variable.
typeThe kind of data to be assigned to a variable. The type used in this lesson is String. Other types, which will be introduced in future lessons, are: boolean, int, double, and char.
Literal A notation for representing a ixed value in source code. Its value cannot be changed as a sketch runs. Literals are often used to initialize variables.
Declaration A programming statement that sets aside memory for a particular type of data and assigns the variable name that will refer to that type.
camel notationA convention for naming variables where words are joined together to form a meaningful phrase to describe what is being assigned. Example of a possible variable in camel notation: lightSignalValue
Initialization The initial value assigned to a newly declared variable.
Assignmentoperator The symbol used in a programming statement to store a value to a variable.The symbol is the equals sign, =.
concatenation The process of appending the value of one String variable to the value of another String variable.